Guide to Object-oriented Programming With Java (University at Buffalo Version)
Chapter 17: Using WindowBuilder to Create GUI Applications
Using WindowBuilder to create a GUI application
There are many different controls that can be added to a frame or panel, including labels, textboxes, buttons, drop-down selectors, check boxes, radio buttons, and sliders, just to list a few.
This next figure shows the code for a ColorPicker program that allows the user to use sliders to determine the RGB (Red/Green/Blue) values for a color.
package buffalo.edu.fa23.put-your-username-here; /** * File: ColorPicker.java * * Description: This app illustrates the use of the * java.awt.Color class. It lets the user type red, green and * blue (RGB) values into text fields, and displays the resulting * color both as a font color and as a colored rectangle. * This version of ColorPicker uses programmer defined IntFields * which throw an out-of-range exception if the values input are * not between 0 and 255. The only changes required occur in the * declarations for the IntFields, in the init() method where the * IntFields are instantiated, and in the actionPerformed() method * the the getInt() method is used to retrieve their data. * * Assignment: * 1) Run this program to see how it works * 2) Modify this program so that it uses JSliders to * select the range of colors. Arrange the JSliders * so they are oriented horizontally and stacked using * a grid layout: * * |-------- slider1 ----------| * |-------- slider2 ----------| * |-------- slider3 ----------| * * - See the example below (SliderExample) to get started */ /** * * @author Jim Gerland * */ import javax.swing.*; import java.awt.*; import javax.swing.event.*; public class ColorPicker extends JFrame implements ChangeListener { private static final long serialVersionUID = 1L; private int redIn, greenIn, blueIn; // create the red slider private final JSlider Red_slider = new JSlider(SwingConstants.HORIZONTAL,0,255,128); // create the green slider private final JSlider Green_slider = new JSlider(SwingConstants.HORIZONTAL,0,255,128); // create the blue slider private final JSlider Blue_slider = new JSlider(SwingConstants.HORIZONTAL,0,255,128); private JPanel controls = new JPanel(); private Canvas canvas = new Canvas(); /** * init() sets up the app's interface. A (default) border layout * is used, with the controls at the north and the drawing canvas * in the center. */ public ColorPicker() { initControls(); getContentPane().add(controls, BorderLayout.NORTH); canvas.setForeground(Color.LIGHT_GRAY); getContentPane().add(canvas, "Center"); canvas.setBorder(BorderFactory.createTitledBorder("The Color Display")); getContentPane().setBackground(Color.white); setSize(300,250); // register 'Exit upon closing' as a default close operation setDefaultCloseOperation( EXIT_ON_CLOSE ); } // end ColorPicker() /** * initControls() arranges the app's control components in a separate * JPanel, which is placed at the north border. The controls consist of * three JTextFields into which the user can type RGB values. */ private void initControls() { // HINT create 3 sliders instead of IntFields controls.setLayout(new GridLayout(0,2)); JLabel R = new JLabel("Red:"); // adds the red slider label to the panel R.setFont(new Font("Tahoma", Font.BOLD, 14)); R.setForeground(new Color(0, 0, 0)); R.setBackground(Color.BLUE); R.setHorizontalAlignment(SwingConstants.RIGHT); controls.add(R); controls.add(Red_slider);//adds the red slider to the panel JLabel G = new JLabel("Green:"); // adds the green slider label to the panel G.setFont(new Font("Tahoma", Font.BOLD, 14)); G.setHorizontalAlignment(SwingConstants.RIGHT); controls.add(G); controls.add(Green_slider); // adds the green slider to the panel JLabel B = new JLabel("Blue:"); // adds the blue slider label to the panel B.setFont(new Font("Tahoma", Font.BOLD, 14)); B.setHorizontalAlignment(SwingConstants.RIGHT); controls.add(B); controls.add(Blue_slider);// adds the blue slider to the panel redIn = Red_slider.getValue(); greenIn = Green_slider.getValue(); blueIn = Blue_slider.getValue(); Red_slider.addChangeListener(this); Green_slider.addChangeListener(this); Blue_slider.addChangeListener(this); } // end initControls() public void stateChanged(ChangeEvent e) { JSlider value = (JSlider)e.getSource(); if (value == Red_slider) { redIn = value.getValue(); canvas.setColor(new Color(redIn,greenIn,blueIn)); } else if(value == Green_slider) { greenIn = value.getValue(); canvas.setColor(new Color(redIn,greenIn,blueIn)); } else if(value == Blue_slider) { blueIn = value.getValue(); canvas.setColor(new Color(redIn,greenIn,blueIn)); } canvas.repaint(); } // end stateChanaged() public static void main(String args[]) { ColorPicker c = new ColorPicker(); c.setVisible(true); } // end main() } // end ColorPicker
ColorPicker.java
Code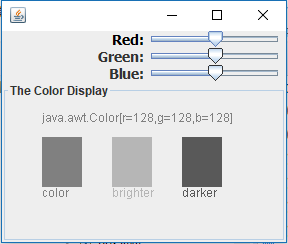
ColorPicker.java
ApplicationLet's get started with a Conversion program